Cookie consent script in CSS and jQuery
Snippets - 21/12/2015
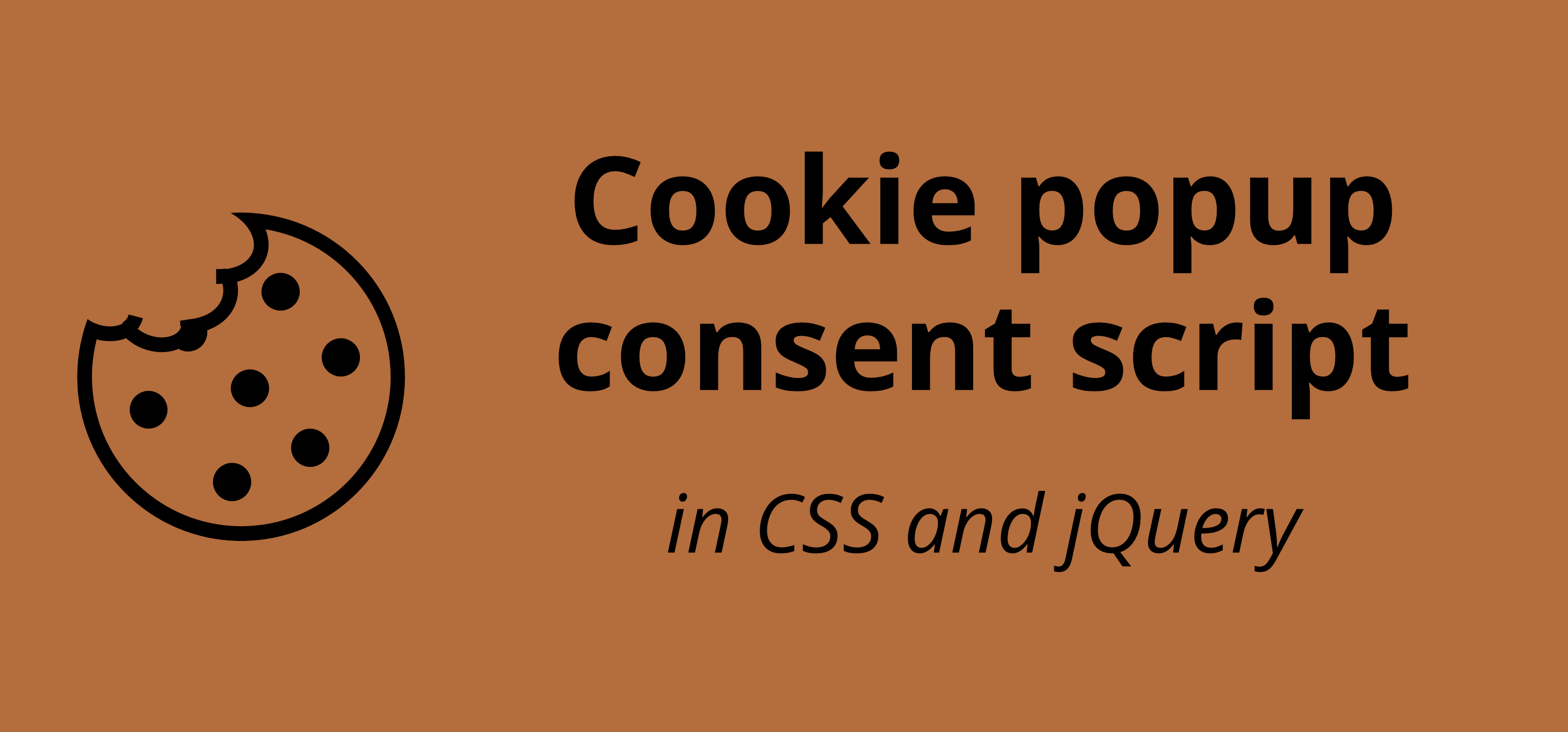
Create a cookie in javascript
In this first part, we'll just create a cookie. To do this, we will use javascript. The cookie validity period is expressed in milliseconds relative to the current date.Here is a sample script to create a cookie
function oft_setCookie(cname) {
// Create a new date
var d = new Date();
// Add the duration of conservation to the current date, it is here 1 year in millisecondes
d.setTime(d.getTime() + 30660000000);
// Create a string to be added in the cookie
document.cookie = cname + "=y; expires=" + d.toUTCString();
}
We can see here that I use the value "y" to see if the cookie is set. You can obviously put what you want, for example a date and time. This eventually allows for additional information.
Check the presence of a cookie
In this third part, we will check if the cookie that we just created exists and is still valid. Indeed, one may have to reapply the consent of the user based on recommendations given by the European Commission.Here is a sample script to check for a cookie
function oft_getCookie(cname) {
// Specifies the name of the portion of the cookie that is sought, the cname is the name of the field
var name = cname + "=";
// Cut the content of the cookie in relation to nature ';'
var ca = document.cookie.split(';');
// Loop on each part of the cookie obtained
for(var i = 0; i < ca.length; i++) {
// Retrieving the current value
var c = ca[i];
// Remove any spaces
while (c.charAt(0) == ' ') c = c.substring(1);
// Returns the value of the field that is sought if it matches
if (c.indexOf(name) == 0) return c.substring(name.length, c.length);
}
return "";
}
Verify the presence of the cookie and its contents
In this fourth part, we will see how to check the presence of a cookie that was created. If the consent is not valid anymore or is absent the consent request message will appear to the user.Here is a sample script to use to show or hide cookie content
function oft_checkCookie() {
// Recovering the content of our value in the cookie
var check = oft_getCookie("oft_cookieConsent");
// If the value is not 'y' the div is displayed with the id "oft_cookieConsent" it is our consent request message
if (check != "y")
// Displaying the consent request in jQuery div
$('#oft_cookieConsent').show();
else
// Hide the request for consent div
$('#oft_cookieConsent').hide();
}
"oft_cookieConsent" is the name I gave the cookie to the request for consent. You can put whatever you want.
Overall function of adding the HTML code, the contents div and listeners
In this part, we will see how to articulate the set of scripts that have been created just before.Here is a sample script for adding the div with text, display or not its content and manage the click validating the consent
function oft_cookieConsent() {
// Add consent solicitation div, hidden at first
$("body").append('<div id="oft_cookieConsent" style="display:none;position:fixed;bottom:0;width:100%;background-color:#999999;padding:5px;color:#FFFFFF;"><div style="display:inline-block;padding-right:20px;">This website uses cookies to ensure you get the best experience on our website <a href="http://online-free-tools.com/en/cookies">More info</a></div><div style="display:inline-block;"><button id="oft_cookieConsent_valid" class="btn btn-primary">Got it!</button></div></div>');
// Displays or not the div that has just been added depending on the presence of a cookie
oft_checkCookie();
// Listen if the user clicks on the accept button cookie
$('#oft_cookieConsent_valid').on('click', function(){
// Create the cookie
oft_setCookie("oft_cookieConsent");
// Hide the div asking for consent
$('#oft_cookieConsent').hide();
});
}
it is possible to customize the content of the div depending on the language of your site. You can simply put a condition check the browser language or go through a global variable created earlier on the page.
Result for the entire script
To summarize, we have seen how to in javascript :
- create a cookie,
- check if a cookie exists,
- get the content of a cookie,
- show or hide a div depending on its value,
- add a div and its content to the HTML code,
- add a listener on the click when giving consent,
Here is a summary of the various pieces of code that we have just seen :
function oft_setCookie(cname) {
var d = new Date();
d.setTime(d.getTime() + 30660000000);
var expires = "expires=" + d.toUTCString();
document.cookie = cname + "=y; " + expires;
}
function oft_getCookie(cname) {
var name = cname + "=";
var ca = document.cookie.split(';');
for(var i = 0; i < ca.length; i++) {
var c = ca[i];
while (c.charAt(0) == ' ') c = c.substring(1);
if (c.indexOf(name) == 0) return c.substring(name.length, c.length);
}
return "";
}
function oft_checkCookie() {
var check = oft_getCookie("oft_cookieConsent");
if (check ! = "y") $('#oft_cookieConsent').show(); else $('#oft_cookieConsent').hide();
}
function oft_cookieConsent() {
$("body").append('<div id="oft_cookieConsent" style="display:none;position:fixed;bottom:0;width:100%;background-color:#999999;padding:5px;color:#FFFFFF;"><div style="display:inline-block;padding-right:20px;">This website uses cookies to ensure you get the best experience on our website <a href="http://online-free-tools.com/en/cookies">More info</a></div><div style="display:inline-block;"><button id="oft_cookieConsent_valid" class="btn btn-primary">Got it!</button></div></div>');
oft_checkCookie();
$('#oft_cookieConsent_valid').on('click', function(){
oft_setCookie("oft_cookieConsent");
$('#oft_cookieConsent').hide();
});
}
oft_cookieConsent();
To go further, it would be interesting to broadcast the message of consent to European users. However it is almost impossible to know for sure. It is possible to go through databases to geolocate the user's IP address, but this information is not secure and is, as the name suggests, that information.
It is important for you to test if the script works in your environment. Do not hesitate if you have any questions.
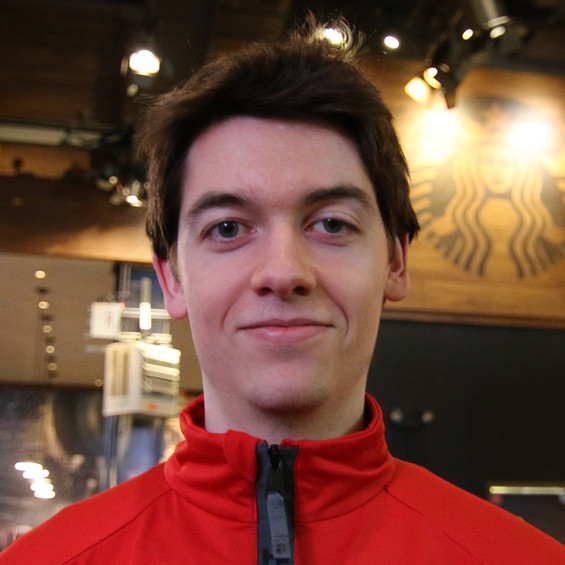
Pierrick CUSSET
Autodidact passionate about the web, I'm always looking for new challenges.
Founder of Online-Free-Tools.com.
Comments
No comment